Automated Trading Bot with MetaTrader5 and Python
There are a ton of signals to analyze when using quantitative analysis for stock/crypto/futures/FOREX trading!
About This Series
This series demonstrates the automated analysis of 8 different market signals.
Using Python 3, Python Pandas, and MetaTrader5, I’ll show you how to calculate 8 common signals.
All code for this tutorial can be found on my GitHub, and I’ve included working code samples throughout (use at your own risk, give me a shout-out if you do).
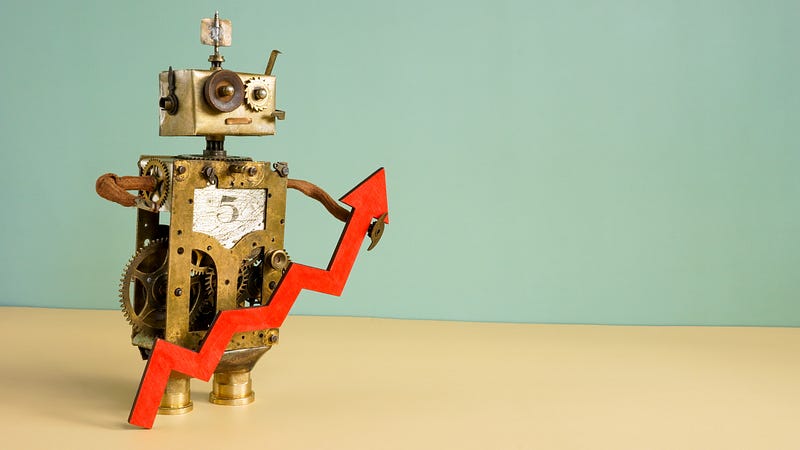
What You Need
Requirements and assumed knowledge as follows:
- Already connected to MetaTrader 5.
- Windows 10 or above. For reasons known only to MetaTrader, the Python API only works on Windows 😊
- Python 3. This series was built with Python 3.10
The Golden Cross
Introduction to the Golden Cross Pattern
In quantitative analysis, the Golden Cross pattern is often used to identify bullish breakouts for a price level. It identifies a moment when a short-term moving average crosses above a longer-term moving average, as shown in the example chart below:
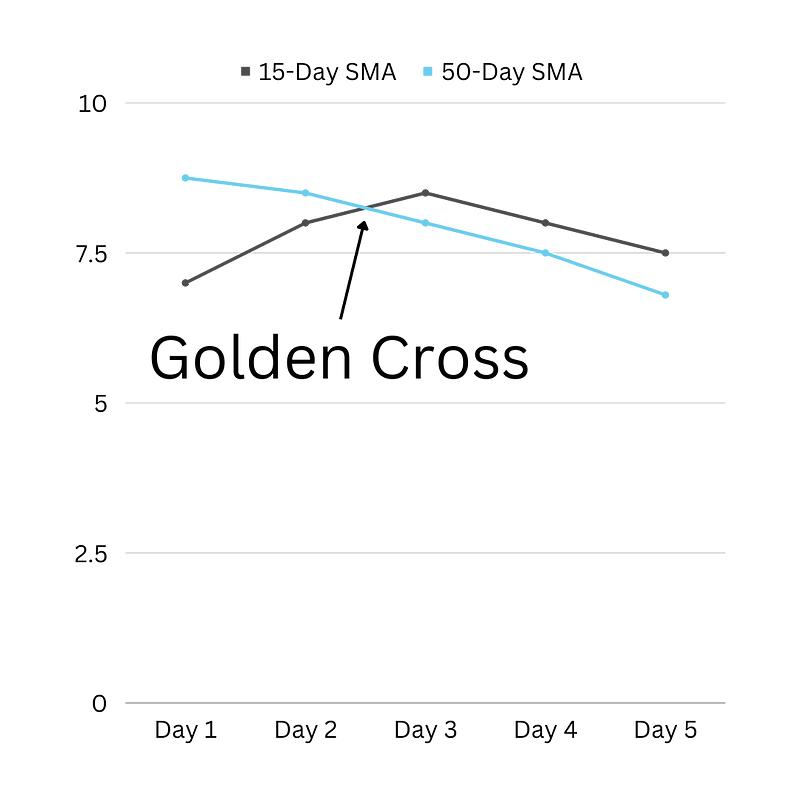
Theoretically, this calculation can be applied to any time-period, however, it is common to use the 15-Day moving average and 50-Day moving average.
How to Calculate
Mathematically, a Golden Cross occurs when the previous short-term moving average is below the previous long-term moving average, followed by the current short-term average moving above the current long-term moving average.
Simplified into pseudo-code:
- Determine the previous 15-day moving average
prev_15
- Determine the previous 50-day moving average
prev_50
- Determine the current 15-day moving average
curr_15
- Determine the current 50-day moving average
curr_50
- If
prev_15 < prev_50
ANDcurr_15 > curr_50
:golden_cross = True
This can be calculated using the Simple Moving Average (SMA) and Exponential Moving Average (EMA).
How to Code
Generic Golden Cross Function
To turn this into code, I’ll start by developing a generic SMA death cross function that can be applied to any timeframe. I’ll assume that we’ll always use the 15 and 200 SMA numbers for comparison (although you can easily change the function to calculate different SMA’s if you wish).
Here’s the code:
import mt5_interface
import pandas
# Function to calculate a death cross event from Moving Averages
def generic_sma_golden_cross_calculator(symbol, timeframe):
# Retrieve 51 rows of timeframe data
raw_data = mt5_interface.query_historic_data(symbol=symbol, timeframe=timeframe, number_of_candles=51)
# Convert raw data into Dataframe
dataframe = pandas.DataFrame(raw_data)
#### Split into required time periods
# Get the previous SMA 15
prev_15_data = dataframe.iloc[-16:-1]
# Calculate the mean
prev_15 = prev_15_data['close'].mean()
# Get the current SMA 15
curr_15_data = dataframe.iloc[-15:]
# Calculate the mean
curr_15 = curr_15_data['close'].mean()
# Get the previous SMA 50
prev_50_data = dataframe.iloc[-51:-1]
# Calculate the mean
prev_50 = prev_50_data['close'].mean()
# Get the current SMA 50
curr_50_data = dataframe.iloc[-50:]
# Calculate the mean
curr_50 = curr_50_data['close'].mean()
# Compare to see if a Cross of Death has occurred
if prev_15 < prev_50 and curr_15 > curr_50:
return True
return False
Note. The file mt5_interface.py
contains the code to connect to MetaTrader.
If you want to run it now in your __main__
here’s how:
# Set up the import filepath
import_filepath = "settings.json"
# Function to import settings from settings.json
def get_project_settings(importFilepath):
# Test the filepath to sure it exists
if os.path.exists(importFilepath):
# Open the file
f = open(importFilepath, "r")
# Get the information from file
project_settings = json.load(f)
# Close the file
f.close()
# Return project settings to program
return project_settings
else:
return ImportError
# Main function
if __name__ == '__main__':
# Import project settings
project_settings = get_project_settings(import_filepath)
# Start MT5
mt5_interface.start_mt5(project_settings["username"], project_settings["password"], project_settings["server"],
project_settings["mt5Pathway"])
mt5_interface.initialize_symbols(project_settings['symbols'])
# Queries
cross_event = generic_golden_cross.generic_sma_golden_cross_calculator(symbol=project_settings['symbols'][0], timeframe="D1")
print(cross_event)
SMA Golden Cross Function
Calculating a ‘classic’ Golden Cross is now as simple as creating a function with the default values. Here’s how:
import generic_golden_cross
# Function to calculate a 'classic' Golden Cross
def calc_sma_golden_cross(symbol):
return generic_golden_cross.generic_sma_golden_cross_calculator(symbol=symbol, timeframe="D1")
Update your import
functions in main.py
then press Play and you should get something like this (note your output may change depending on when you do this):
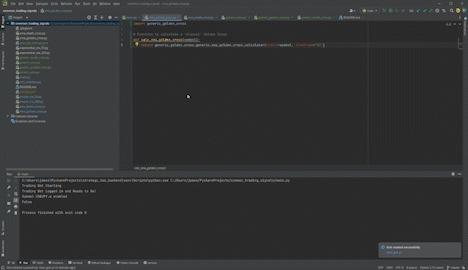
Wrapping Up
And that’s how you calculate a Golden Cross calculation into your Python Trading Bot. Let me know in the comments how you’re planning to use it!
Say Hi!
I love hearing from my readers, so feel free to reach out. It means a ton to me when you clap for my articles or drop a friendly comment — it helps me know that my content is helping.
❤