Automated Trading Bot with MetaTrader5 and Python
There are a ton of signals to analyze when using quantitative analysis for stock/crypto/futures/FOREX trading!
About This Series
This series demonstrates the automated analysis of 8 different market signals.
Using Python 3, Python Pandas, and MetaTrader5, I’ll show you how to calculate 8 common signals.
All code for this tutorial can be found on my GitHub, and I’ve included working code samples throughout (use at your own risk, give me a shout-out if you do).

What You Need
Requirements and assumed knowledge as follows:
- Already connected to MetaTrader 5.
- Windows 10 or above. For reasons known only to MetaTrader, the Python API only works on Windows 😊
- Python 3. This series was built with Python 3.10
The Death Cross
Introduction to the Cross of Death
The ‘Cross of Death’ (aka Death Cross) is a stock market chart pattern reflecting recent price weakness. In generic terms, it occurs when a shorter-term moving average drops below a longer-term moving average.
For SMA stock calculations, these time periods are frequently the 50-day moving average and 200-day moving average. I.e. a death cross occurs when the 50-day moving average drops below the 200-day moving average. Visually this might look like this:
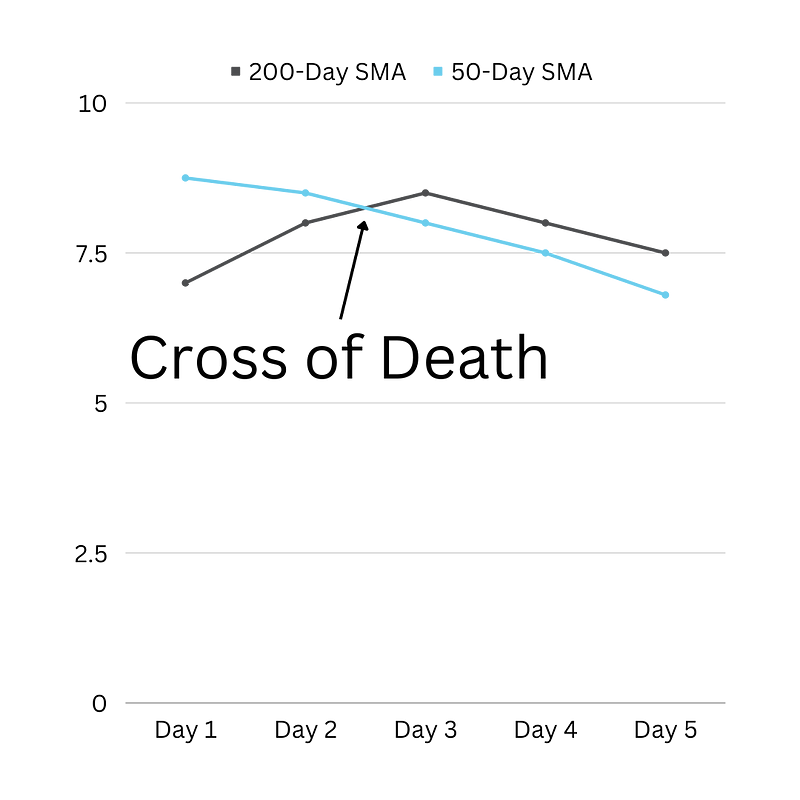
How to Calculate
A Death Cross occurs mathematically when the previous short-term moving average is above the previous long-term moving average and the current short-term moving average is below the current long-term moving average.
Simplified into pseudo-code:
- Determine the previous 50-day moving average
prev_50
- Determine the previous 200-day moving average
prev_200
- Determine the current 50-day moving average
curr_50
- Determine the current 200-day moving average
curr_200
- If
prev_50 > prev_200
ANDcurr_50 < curr_200
:cross_of_death = True
This can be calculated using the Simple Moving Average (SMA) and Exponential Moving Average (EMA).
How to Code
Generic Death Cross Function
To turn this into code, I’ll start by developing a generic SMA death cross function that can be applied to any timeframe. I’ve assumed that we’ll use a 50 and 200 SMA for comparison (although you could change the function to calculate different SMAs).
Here’s the code:
import mt5_interface
import pandas
# Function to calculate a death cross event from Moving Averages
def generic_sma_death_cross_calculator(symbol, timeframe):
# Retrieve 201 rows of timeframe data
raw_data = mt5_interface.query_historic_data(symbol=symbol, timeframe=timeframe, number_of_candles=201)
#### Split into required time periods
# Convert raw data into Dataframe
dataframe = pandas.DataFrame(raw_data)
# Get the previous SMA 50
prev_50_data = dataframe.iloc[-51:-1]
# Calculate the mean
prev_50 = prev_50_data['close'].mean()
# Get the current SMA 50
curr_50_data = dataframe.iloc[-50:]
# Calculate the mean
curr_50 = curr_50_data['close'].mean()
# Get the previous SMA 200
prev_200_data = dataframe.iloc[-201:-1]
# Calculate the mean
prev_200 = prev_200_data['close'].mean()
# Get the current SMA 200
curr_200_data = dataframe.iloc[-200:]
# Calculate the mean
curr_200 = curr_200_data['close'].mean()
# Compare to see if a Cross of Death has occurred
if prev_50 > prev_200 and curr_50 < curr_200:
return True
return False
Note. The file mt5_interface.py
contains the code to connect to MetaTrader.
If you want to run it now in your __main__
here’s a Gist of my main.py
:
import json
import os
import mt5_interface
import generic_death_cross
# Set up the import filepath
import_filepath = "settings.json"
# Function to import settings from settings.json
def get_project_settings(importFilepath):
# Test the filepath to sure it exists
if os.path.exists(importFilepath):
# Open the file
f = open(importFilepath, "r")
# Get the information from file
project_settings = json.load(f)
# Close the file
f.close()
# Return project settings to program
return project_settings
else:
return ImportError
# Main function
if __name__ == '__main__':
# Import project settings
project_settings = get_project_settings(import_filepath)
# Start MT5
mt5_interface.start_mt5(project_settings["username"], project_settings["password"], project_settings["server"],
project_settings["mt5Pathway"])
mt5_interface.initialize_symbols(project_settings['symbols'])
# Queries
cross_event = generic_death_cross.generic_sma_death_cross_calculator(symbol=project_settings['symbols'][0], timeframe="D1")
print(cross_event)
SMA Death Cross Function
Abstracting the generic SMA Death Cross function means determining if a Cross of Death has occurred in the last day is only a few lines:
import generic_death_cross
# Function to calculate a Death Cross event
def calc_sma_death_cross(symbol):
return generic_death_cross.generic_sma_death_cross_calculator(symbol=symbol, timeframe="D1")
If you update your __main__
to reference this new function, then run it, you should get a boolean True
or False
like I have below (your results will vary based on when you do it and what symbol you use):
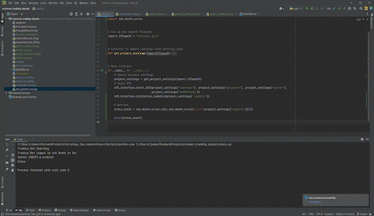
Wrapping Up
As they say, ‘That’s a Wrap!’ I hope this helps your trading journey — let me know how it goes in the comments below.
Say Hi!
I love hearing from my readers, so feel free to reach out. It means a ton to me when you clap for my articles or drop a friendly comment — it helps me know that my content is helping.
❤